Dynamic Smooth type Reveal Animation
Webflow
Smooth text reveal animation in just few steps, attribute solution. Smooth Type Reveal Animations to Captivate Audiences

1. Importing SplitType and gsap
To access SplitType and GSAP via CDN, first, locate their respective CDN URLs on a service like jsDelivr.
For GSAP, you can find the URL by visiting the GSAP website or searching for "GSAP CDN" online. Once you have the GSAP CDN URL, you can include it in your HTML file within a script tag.
Similarly, for SplitType, locate its CDN URL and include it in another script tag in your HTML file.
Make sure to place these script tags before your custom JavaScript code to ensure that SplitType and GSAP are available when your script runs. Once included, you can start using the functionality provided by SplitType and GSAP in your project.
<script src="https://unpkg.com/split-type"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/gsap.min.js"></script>
2. Add this Code before closing /body tag
3. Add the target class to the html
We are targeting a class called typed_js in this code. In our heading class, we've added the class by adding a span. One thing to note is that for the animation to work properly, we have to wrap it in a div which needs to have a fixed height and a relative position. This is because we are emptying and appending that span's element, positioning them absolutely within the wrapper. For more information, please check out the walkthrough video on YouTube.
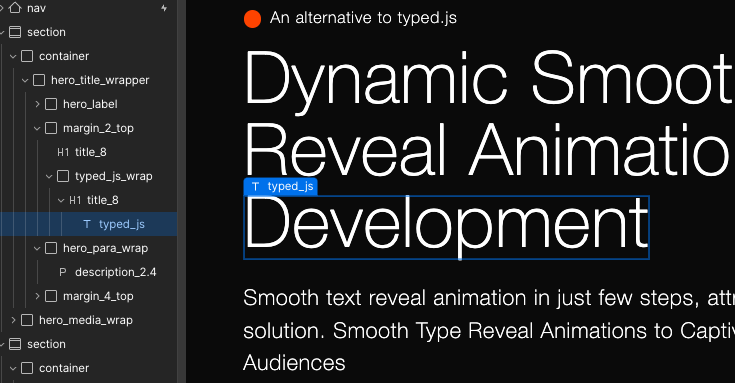